Screen Capture
The Screen Capture feature allows users to take screenshots within your application and ask questions about specific UI elements or issues. This guide will walk you through the implementation and usage.
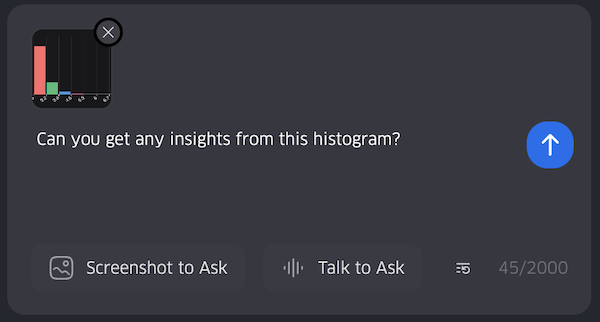
Installation
npm install @openassistant/ui
Usage
First, wrap your application with the ScreenshotWrapper
component:
import { AiAssistant, ScreenshotWrapper } from '@openassistant/ui';
import { useState } from 'react';
function App() {
const [startScreenCapture, setStartScreenCapture] = useState(false);
const [screenCaptured, setScreenCaptured] = useState('');
return (
<ScreenshotWrapper
setScreenCaptured={setScreenCaptured}
startScreenCapture={startScreenCapture}
setStartScreenCapture={setStartScreenCapture}
>
<YourAppContent />
</ScreenshotWrapper>
);
}
Integrate the AI Assistant component with screen capture capabilities by setting the following props:
enableScreenCapture
: Enable screen capturescreenCapturedBase64
: The base64 encoded screenshotonScreenshotClick
: The callback function when the user clicks the screenshot buttononRemoveScreenshot
: The callback function when the user removes the screenshot
<AiAssistant
modelProvider="openai"
model="gpt-4"
apiKey={process.env.OPENAI_API_KEY}
welcomeMessage="How can I assist you today?"
enableScreenCapture={true}
screenCapturedBase64={screenCaptured}
onScreenshotClick={() => setStartScreenCapture(true)}
onRemoveScreenshot={() => setScreenCaptured('')}
/>
User Guide
Taking Screenshots
- Click the "Screenshot to Ask" button in the chat interface
- A semi-transparent overlay will appear
- Click and drag to select the area you want to capture
- Release to complete the capture
Asking Questions
- After capturing, the screenshot will be attached to your next message
- Type your question about the captured area
- Send your message to get AI assistance
Managing Screenshots
- Click the "X" button on the screenshot preview to remove it
- Use
onRemoveScreenshot
callback for programmatic removal
Other Configuration
You can save the screenshot to a file by setting the saveScreenshot
prop to true
.
<ScreenshotWrapper
...
setScreenCaptured={setScreenCaptured}
startScreenCapture={startScreenCapture}
setStartScreenCapture={setStartScreenCapture}
saveScreenshot={false} // Enable automatic download
/>